Introduction Introduction
We can add custom image sizes with function add_image_size(). But, First, let’s discuss why we need custom images in WordPress?
By default, WordPress provides 3 image sizes. Means, When we upload any image in WordPress media library then WordPress creates 3 image sizes for us.
These image sizes are:
- Thumbnail
(150x150)
- Medium
(300x300)
- Large
(1024x1024)
Let’s see the image sizes with one example.
I was uploaded below the original image and WordPress create 3 images as below:
Original Image
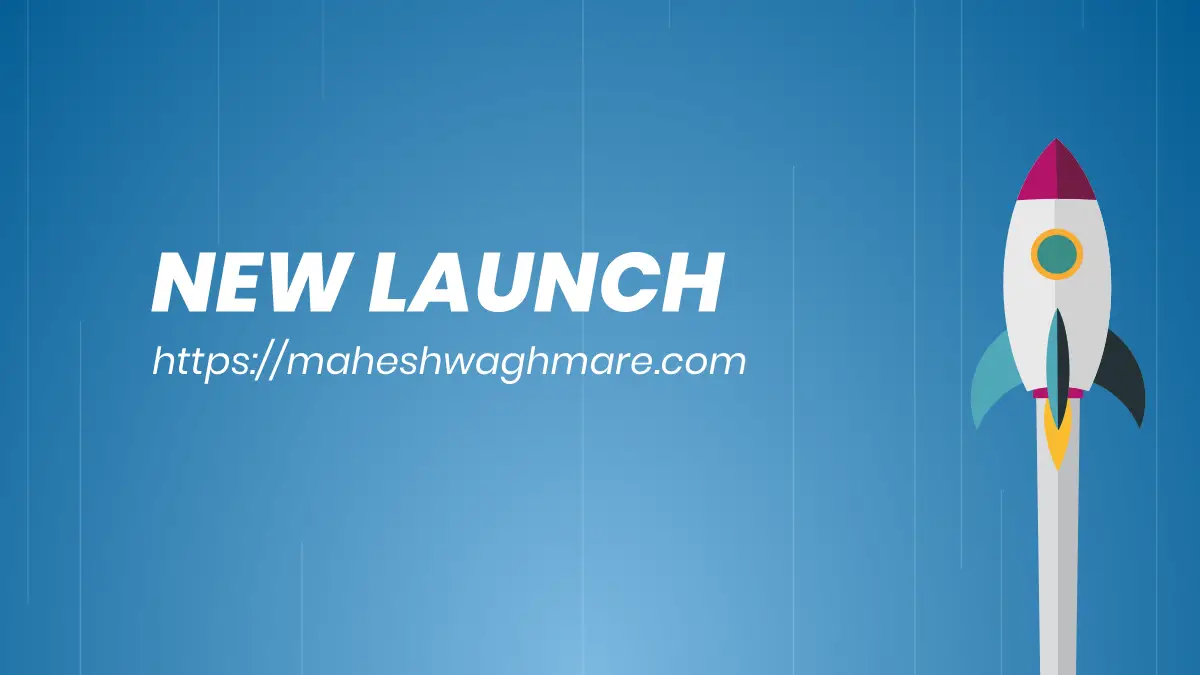
Thumbnail Image
Below is the thumbnail image with a 150×150 resolution.
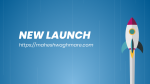
Medium Image
Below is the medium image with a 300×300 resolution.
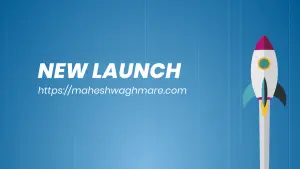
Large Image
Below is the large image with a 1024×1024 resolution.
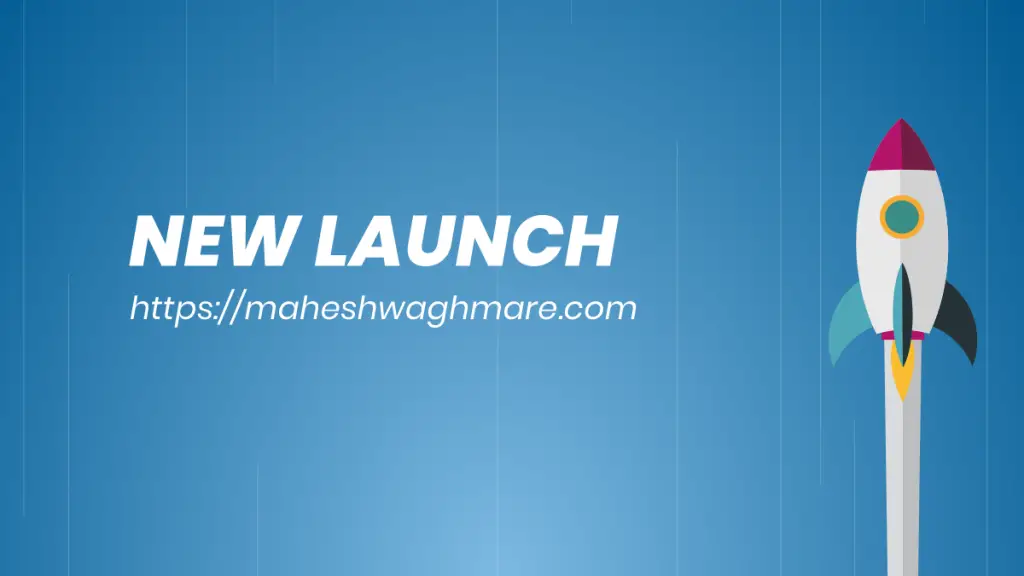
Change Default Image Sizes Change Default Image Sizes
WordPress allows us to change the default image sizes. Now, We know that we have 3 image sizes which are automatically created when an image is uploaded with the media library.
But, If you want to change Thumbnail, Medium and Large image size resolutions then we can easily do it from the Settings > Media
page.
Below is the screenshot of the Media Settings page.
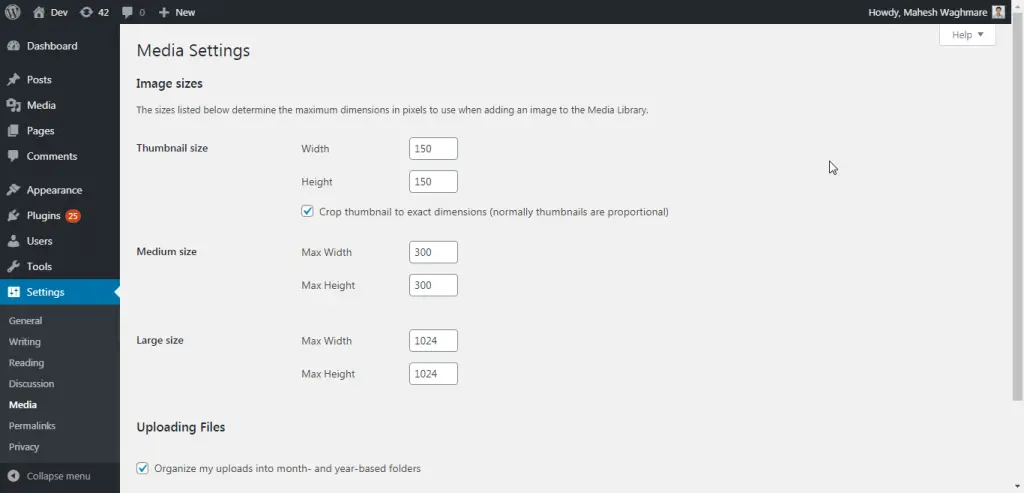
Add Custom Sizes Add Custom Sizes
Now, maybe you have some questions:
- When WordPress provides some default sizes then why we could not use them?
- Why we need custom image sizes?
Let me explain, WordPress provides thumbnail, medium and large image sizes as 150×150, 300×300 and 1024×1024 respectively.
If we have some custom post types and its layout require different image size except above default one. For example, we have a book
post type and we want to show the image 600×600 for the book featured image.
Now, You think that why not to change the medium image size from 300×300 with 600×600?
The reason is, For the book featured image we want 600×600 but it also changes for other post types too.
If we change the medium image size applied for all the uploaded images.
So, the best way is to register a new image size with function add_image_size().
Let’s add 600×600 custom image size with below code snippet:
/** * Add Image Sizes * * @since 1.0.0 */ if( ! function_exist( 'prefix_after_setup_theme' ) ) : function prefix_after_setup_theme() { add_image_size( 'books-featured-image', 600, 600 ); } add_action( 'after_setup_theme', 'prefix_after_setup_theme', 10 ); endif;
Here, We have used action after_setup_theme
to register our books featured image.
The function add_image_size() we have pass 3 values:
books-featured-image
this is an image size name600
this 2nd parameter set the default image width600
this 3rd parameter set the default image height
Note: The function add_image_size() accepts the 4th parameter which is for cropping the image. For now, we don’t want it.
Experiment With Different Sizes Experiment With Different Sizes
Below are some examples of how the image sizes work after passing different parameters.
add_image_size( 'image-size-20', '20' );

add_image_size( 'image-size-20', '20' );
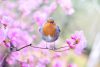
add_image_size( 'image-size-400', '400' );
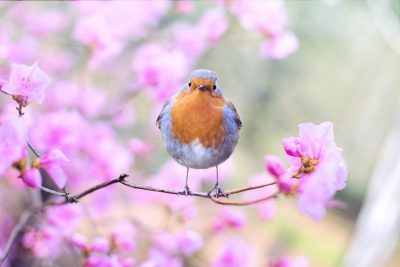
add_image_size( '600-800', '600', '800' );
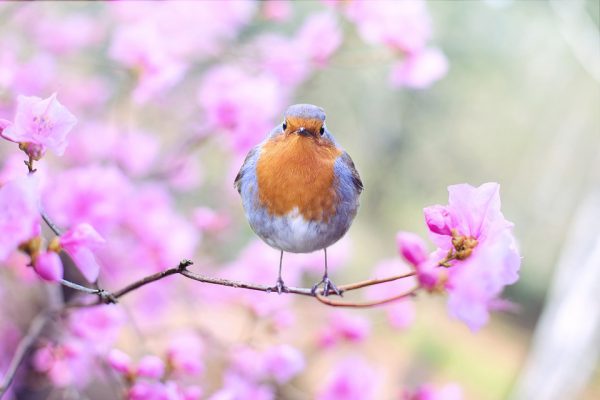
Now with two dimentions
add_image_size( '600-800-true', '600', '800', true );
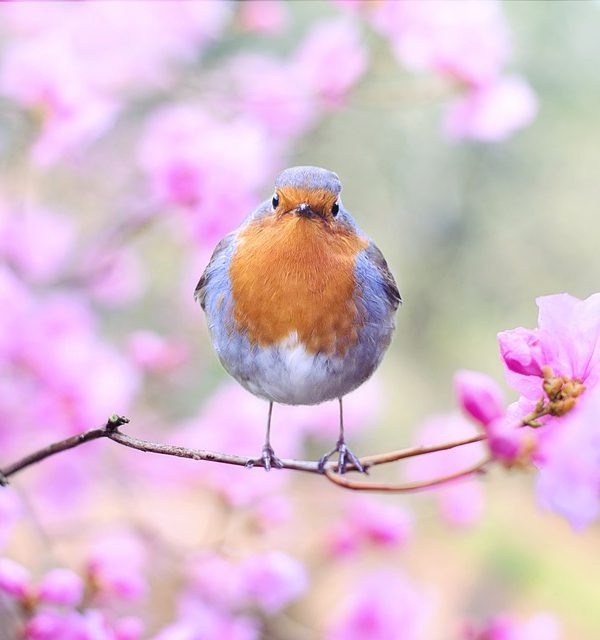
add_image_size( '600-800-left', '600', '800', array( 'left' ) );
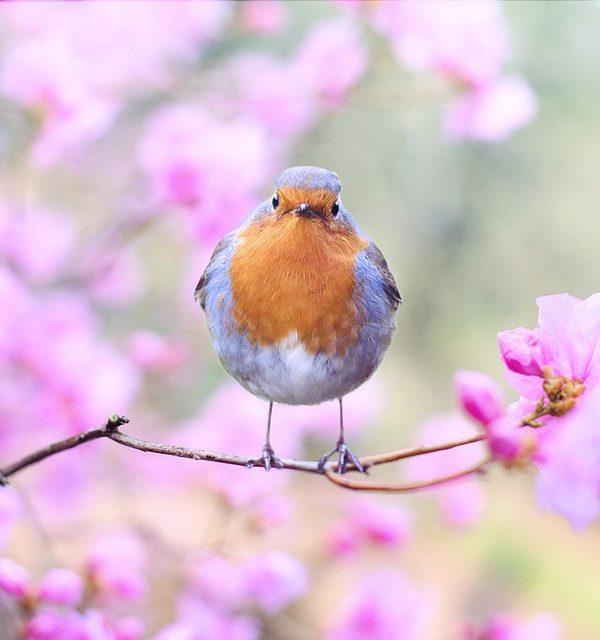
add_image_size( '600-800-center', '600', '800', array( 'center' ) );
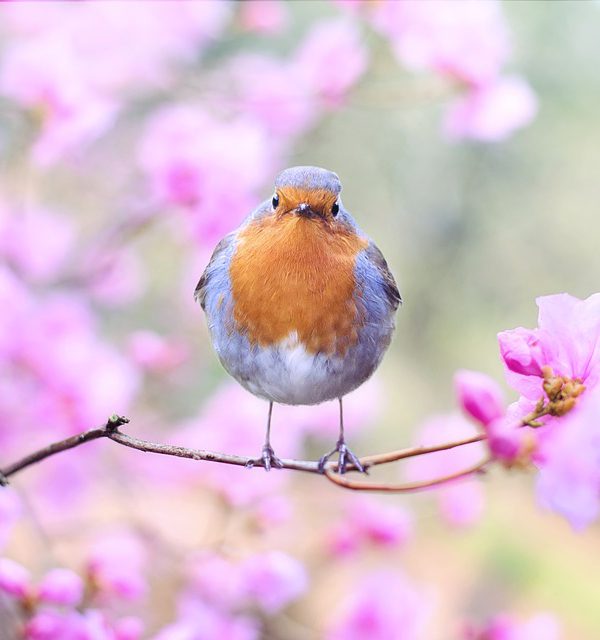
add_image_size( '600-800-right', '600', '800', array( 'right' ) );
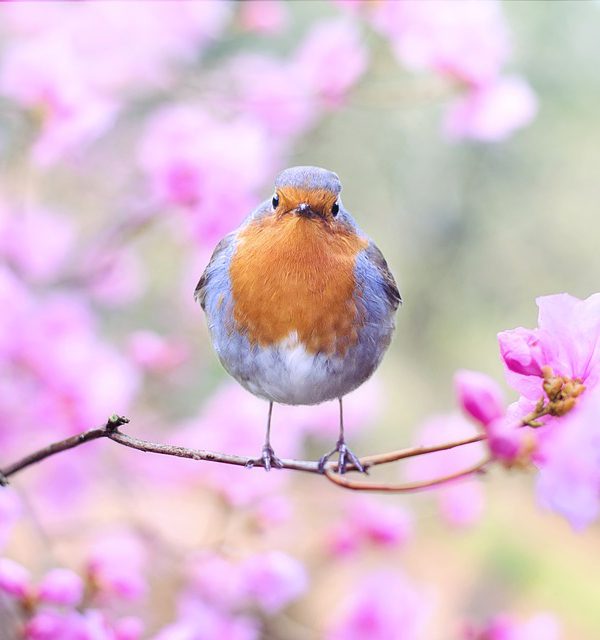
add_image_size( '600-800-left-top', '600', '800', array( 'left', 'top' ) );
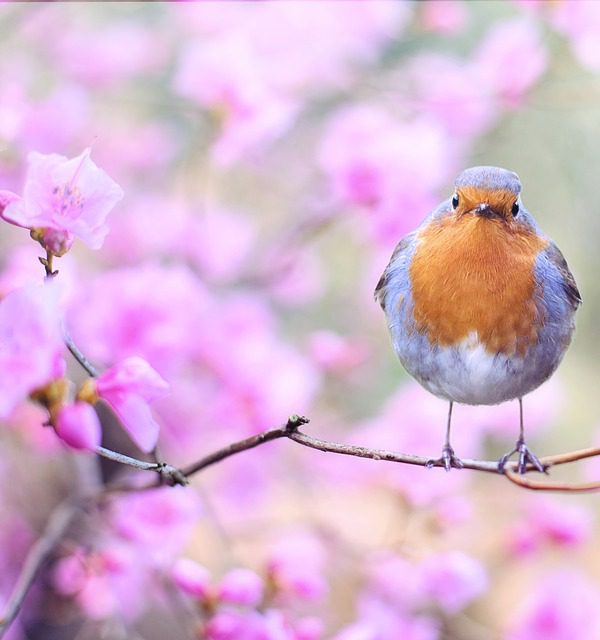
add_image_size( '600-800-center-top', '600', '800', array( 'center' ), 'top' );
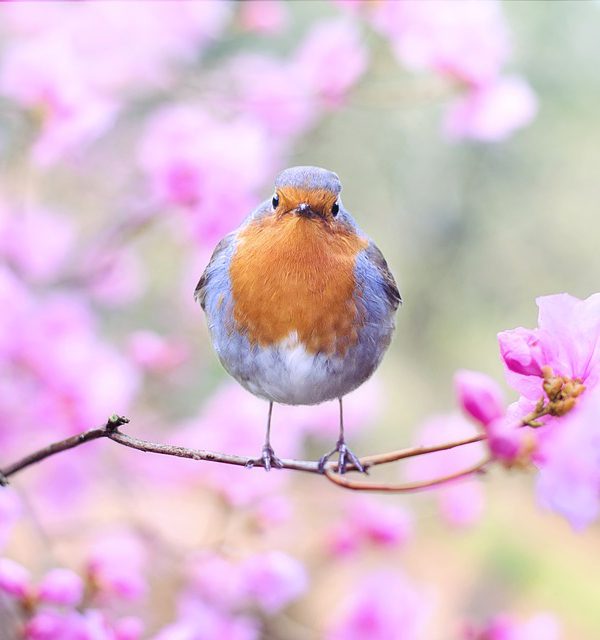
add_image_size( '600-800-right-top', '600', '800', array( 'right' ), 'top' );
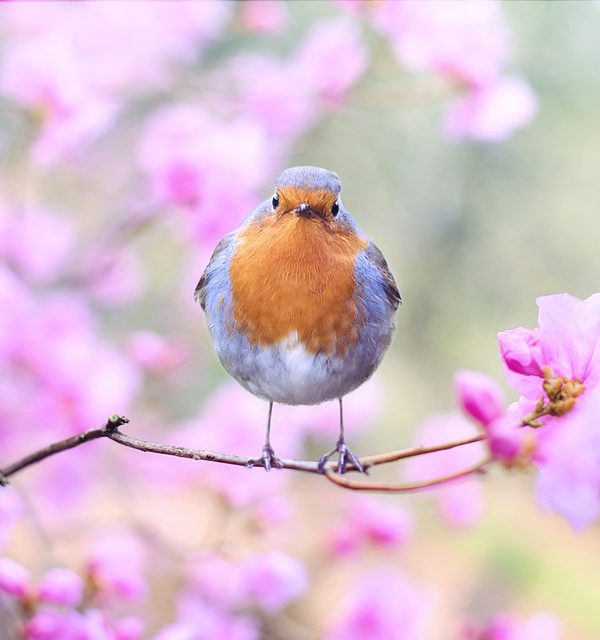
add_image_size( '600-800-left-center', '600', '800', array( 'left', 'center' ) );
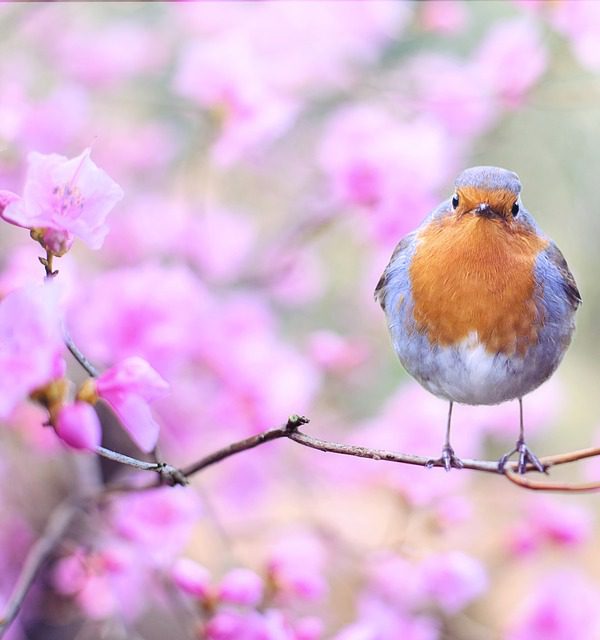
add_image_size( '600-800-center-center', '600', '800', array( 'center' ), 'center' );
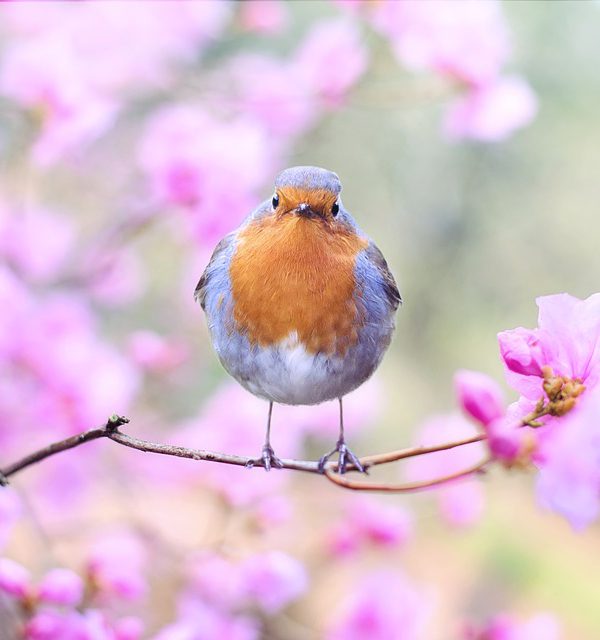
add_image_size( '600-800-right-center', '600', '800', array( 'right' ), 'center' );
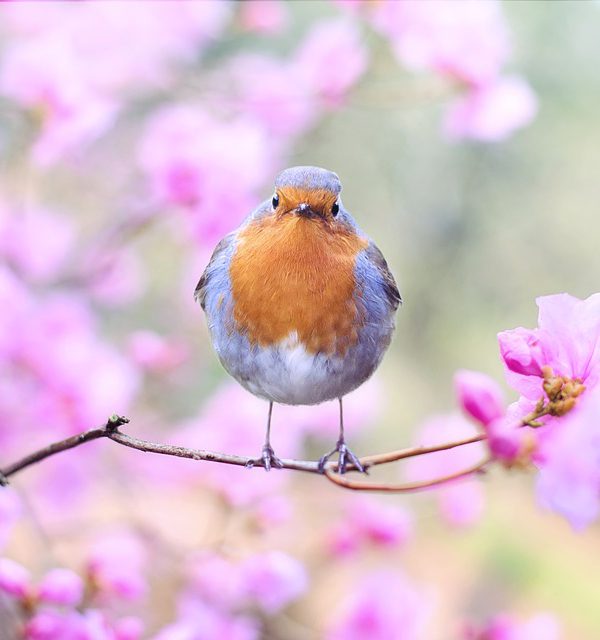
add_image_size( '600-800-left-bottom', '600', '800', array( 'left', 'bottom' ) );
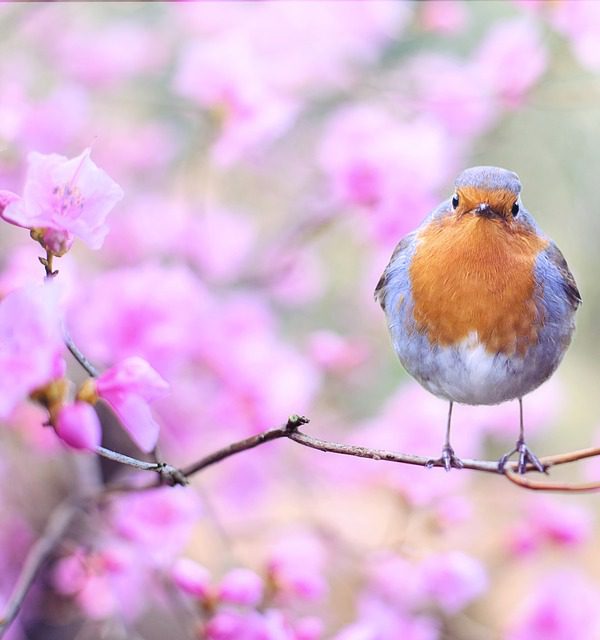
add_image_size( '600-800-center-bottom', '600', '800', array( 'center' ), 'bottom' );
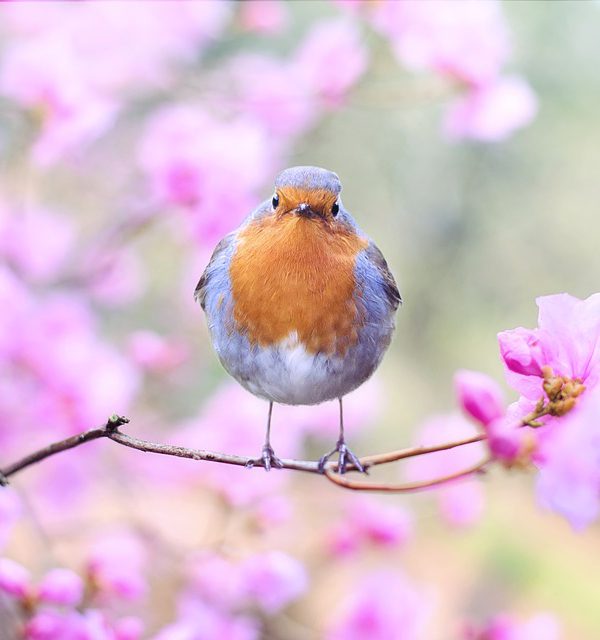
add_image_size( '600-800-right-bottom', '600', '800', array( 'right' ), 'bottom' );
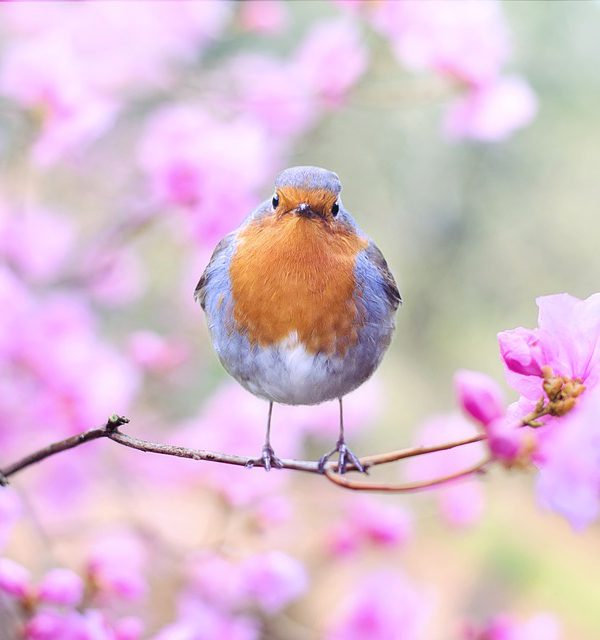
Retrieve Custom Image Size Retrieve Custom Image Size
Now, We are able to get this image size anywhere.
Suppose we want to get our new image size in single book template then we can use below code snippet:
the_post_thumbnail( 'books-featured-image' );
Another example is, Suppose we know the uploaded image ID and we want to get the image size by attachment id then we can use below code snippet:
echo wp_get_attachment_image( 4259, 'books-featured-image' );
Here, The 4259
is an attachment ID.
Regenerate Images Regenerate Images
Suppose, You have already uploaded a lot of images and you want to switch them. And that theme has defined some custom image sizes.
We know that after defining the custom image size if we upload any new image then our custom image size will create.
But, We already have a lot of images that have our custom image size. So, How to create our new defined custom image sizes?
We have a FREE plugin Regenerate Thumbnails which resolve this problem.
After installing the plugin we can regenerate the images with one click.
Also, If you have the WP CLI setup then you can also auto-generate the images with the WP CLI command.
# Regenerate thumbnails for given attachment IDs. $ wp media regenerate 123 124 125 Found 3 images to regenerate. 1/3 Regenerated thumbnails for "Vertical Image" (ID 123). 2/3 Regenerated thumbnails for "Horizontal Image" (ID 124). 3/3 Regenerated thumbnails for "Beautiful Picture" (ID 125). Success: Regenerated 3 of 3 images. # Regenerate all thumbnails, without confirmation. $ wp media regenerate --yes Found 3 images to regenerate. 1/3 Regenerated thumbnails for "Sydney Harbor Bridge" (ID 760). 2/3 Regenerated thumbnails for "Boardwalk" (ID 757). 3/3 Regenerated thumbnails for "Sunburst Over River" (ID 756). Success: Regenerated 3 of 3 images. # Re-generate all thumbnails that have IDs between 1000 and 2000. $ seq 1000 2000 | xargs wp media regenerate Found 4 images to regenerate. 1/4 Regenerated thumbnails for "Vertical Featured Image" (ID 1027). 2/4 Regenerated thumbnails for "Horizontal Featured Image" (ID 1022). 3/4 Regenerated thumbnails for "Unicorn Wallpaper" (ID 1045). 4/4 Regenerated thumbnails for "I Am Worth Loving Wallpaper" (ID 1023). Success: Regenerated 4 of 4 images. # Re-generate only the thumbnails of "large" image size for all images. $ wp media regenerate --image_size=large Do you really want to regenerate the "large" image size for all images? [y/n] y Found 3 images to regenerate. 1/3 Regenerated "large" thumbnail for "Sydney Harbor Bridge" (ID 760). 2/3 No "large" thumbnail regeneration needed for "Boardwalk" (ID 757). 3/3 Regenerated "large" thumbnail for "Sunburst Over River" (ID 756). Success: Regenerated 3 of 3 images.