WordPress Theme Review team release the Webfonts Loader to download the web fonts like Google fonts and host them locally.
We are going to see:
Why to download CDN fonts? Why to download CDN fonts?
According to current WordPress Theme review guidelines themes prohibit the use of CDNs to load assets due to privacy/tracking concerns.
The only exception to that rule is Google Fonts.
To avoid the CDN links like Google fonts we can now use the function wptt_get_webfont_styles() to load the webfonts fonts.
NOTE: It works for any web font including Google Fonts. Here, I’m showing the demo for Google fonts.
How do we load the Google fonts in WordPress? How do we load the Google fonts in WordPress?
// Load theme CORE css.
wp_enqueue_style( 'my-theme', get_stylesheet_directory_uri() . '/style.css', array(), '1.0', 'all' );
// Load fonts from Google.
wp_enqueue_style( 'my-theme-google-fonts', 'https://fonts.googleapis.com/css?family=Open+Sans&400,400i,600,700,800,900&subset=latin,latin-ext', array(), null );
OR
// Load theme CORE css.
wp_enqueue_style( 'my-theme', get_stylesheet_directory_uri() . '/style.css', array(), '1.0', 'all' );
// Load fonts from Google.
wp_enqueue_style( 'my-theme-google-fonts', my_theme_google_fonts_url(), array(), null );
// Genereate and return the Google fonts url.
function my_theme_google_fonts_url() {
$fonts_url = '';
$font_families = array(
'Open Sans:400,400i,600,700,800,900',
);
$query_args = array(
'family' => urlencode( implode( '|', $font_families ) ),
'subset' => urlencode( 'latin,latin-ext' ),
);
$fonts_url = add_query_arg( $query_args, "//fonts.googleapis.com/css" );
return $fonts_url;
}
Both work the same.
But, When I check it with Google Page Speed Insights then I found that it is a render-blocking asset that affects performance.
So, What to do?
We can download the fonts from Google and host them on our website.
It’s pretty simple with a webfont-loader PHP class.
Below is the screenshot of my performance before using the webfont-loader.
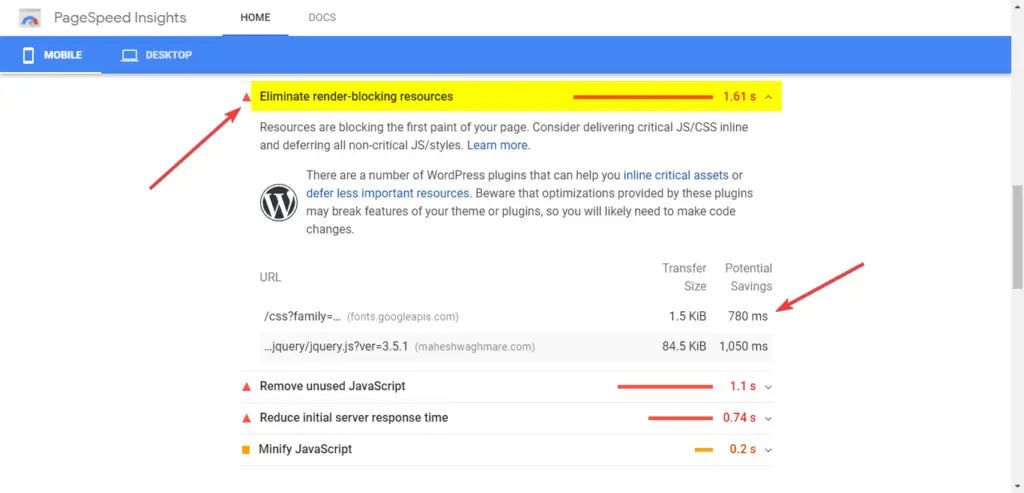
How to use the wptt_get_webfont_styles() function? How to use the wptt_get_webfont_styles() function?
Same as the typical example we can load the web fonts as below:
// Load theme CORE css.
wp_enqueue_style( 'my-theme', get_stylesheet_directory_uri() . '/style.css', array(), '1.0', 'all' );
// Load fonts from Google.
wp_enqueue_style( 'my-theme-google-fonts', wptt_get_webfont_styles('https://fonts.googleapis.com/css?family=Open+Sans&400,400i,600,700,800,900&subset=latin,latin-ext'), array(), null );
OR
Add the web-fonts with wp_add_inline_style() to load them inline.
// Load theme CORE css.
wp_enqueue_style( 'my-theme', get_stylesheet_directory_uri() . '/style.css', array(), '1.0', 'all' );
// Load fonts from Google.
wp_add_inline_style( 'my-theme-google-fonts', wptt_get_webfont_url('https://fonts.googleapis.com/css?family=Open+Sans&400,400i,600,700,800,900&subset=latin,latin-ext') );
You can use any one of them as you want.
For more details visit https://github.com/WPTT/webfont-loader/
After using the webfont-loader performance improved:
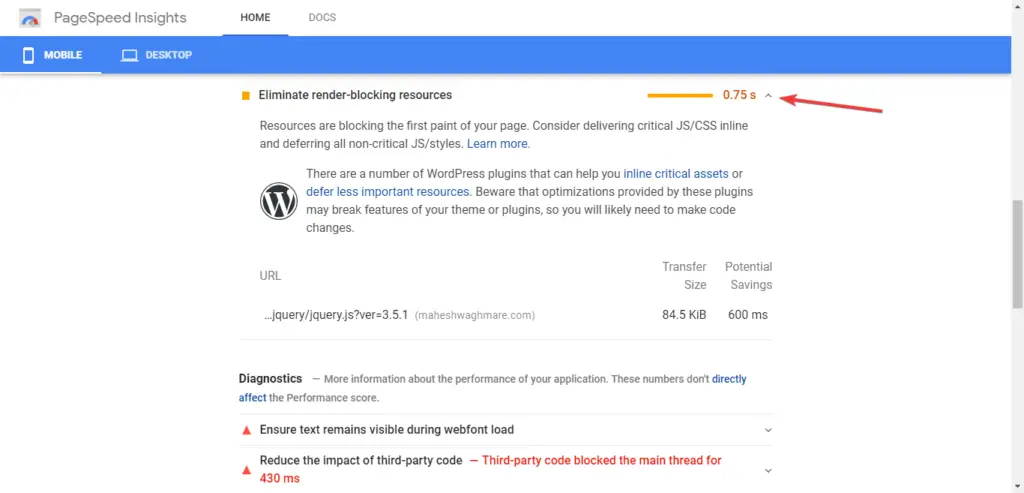
Does it fix the Google Page Speed inside render-blocking asset error? Does it fix the Google Page Speed inside render-blocking asset error?
Yes. See the below comparison:
- Before – 1.5kb / 780ms blocking
- After – 0 blocking for Google Fonts request it means we have 1.5kb size + 780 ms blocking time
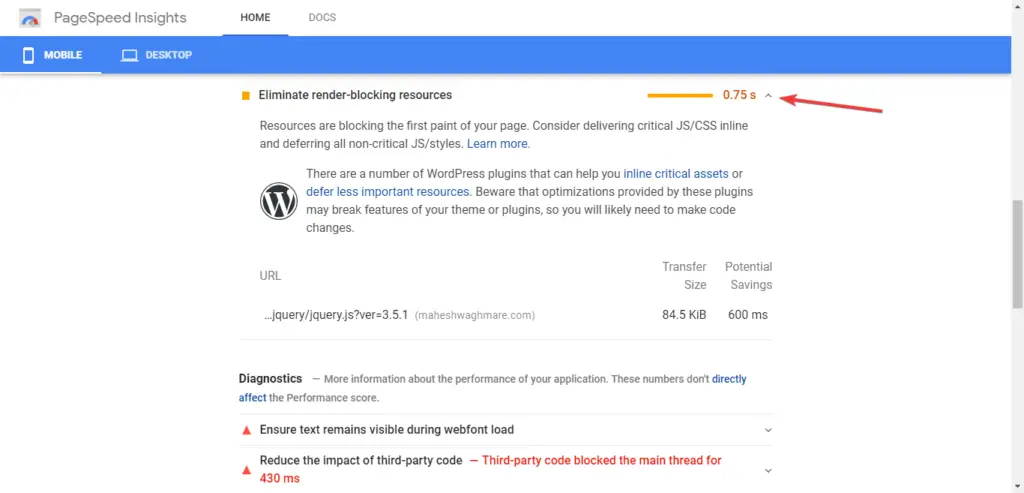
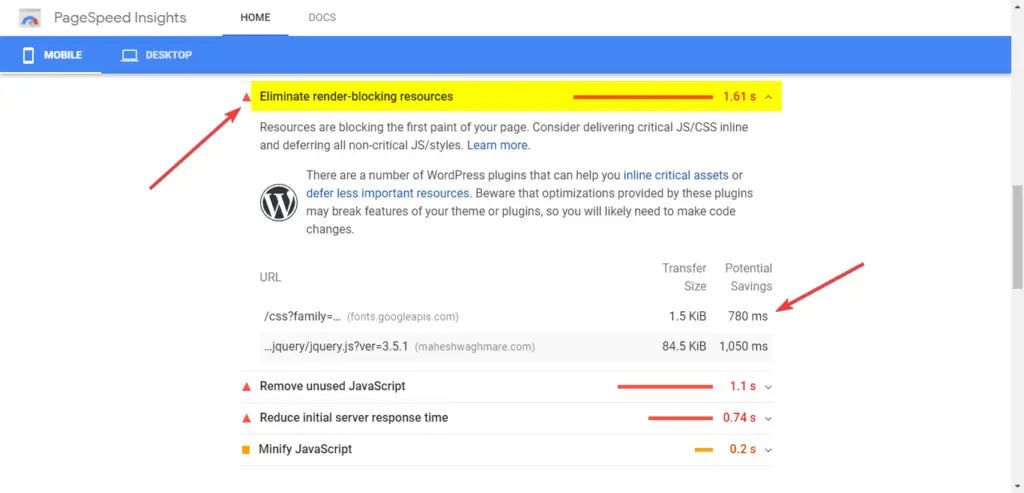
NOTE: In the screenshot, we see the 600ms blocking time which is the jQuery. We optimize the time for the Google Fonts only.