Working on Rest API and OAuth is very interesting. Working on Rest API and OAuth is very interesting.
WordPress merged Rest API support into the core in WordPress 4.7 “Vaughan” check out the Rest API Merge Proposal.
In this post, I’m creating the post on my site https://maheshwaghmare.com/ though rest API and OAuth.
Let’s Try it Step by Step Let’s Try it Step by Step
Step 1: Step 1:
Create new app wordpress.com app from https://developer.wordpress.com/apps/new/
OR
Do you already have an app then select existing app from https://developer.wordpress.com/apps/
Step 2: Step 2:
Copy below code and add your app authentication details. After adding details you get and auth key.
// Step: 1 Add authentication details to get auth key. $auth_args = array( 'username' => '', 'password' => '', 'client_id' => '', 'client_secret' => '', 'grant_type' => 'password', // Keep this as it is. ); $access_key = get_access_key( $auth_args );
Note: Keep grant_type
as password
. Its required!
username
– Your website username.password
– Your website password.client_id
– Your App client ID.client_id
– Your App client ID.
Step 3: Step 3:
Use below function which return the auth key from your given app details.
/** * Get Access Key. * * @param array $args Auth arguments. * @return mixed Auth response. */ function get_access_key( $args ) { // Access Token. $curl = curl_init( 'https://public-api.wordpress.com/oauth2/token' ); curl_setopt( $curl, CURLOPT_POST, true ); curl_setopt( $curl, CURLOPT_POSTFIELDS, $args ); curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1); $auth = curl_exec( $curl ); $auth = json_decode($auth); // Access Key. return $auth->access_token; }
Step 4: Step 4:
Add your new post details. Such as post title, post content etc. like below:
// Step: 2 Set post arguments and pass it create the post. $post_args = array( 'title' => 'Hello APA World', 'content' => 'Hello. I am a test post. I was created by the API', 'tags' => 'tests', 'post_status' => 'draft', 'categories' => 'API', );
Step 5: Step 5:
Now, Simply call a function and pass the Auth key and post arguments.
// Create a post with the access key. create_post( $access_key, $post_args );
Step 6: Step 6:
/** * Create post with access key. * * @param string $access_key Access key. * @param array $post_args Post arguments. * @return mixed Post response. */ function create_post( $access_key, $post_args ) { $options = array ( 'http' => array( 'ignore_errors' => true, 'method' => 'POST', 'header' => array( 0 => 'authorization: Bearer ' . $access_key, 1 => 'Content-Type: application/x-www-form-urlencoded', ), 'content' => http_build_query( $post_args ), ), ); $context = stream_context_create( $options ); $response = file_get_contents( 'https://public-api.wordpress.com/rest/v1/sites/YOURSITEID/posts/new/', false, $context ); return json_decode( $response ); }
Here,
https://public-api.wordpress.com/rest/v1/sites/YOURSITEID/posts/new/
Replace YOURSITEID with your wordpress.com site ID.
To get it follow below quick simple steps:
- Goto api console
- Add string
/me/sites
and select method GET - Press Enter.
It’ll show all your sites from WordPress.com. Check the below screenshot for reference.
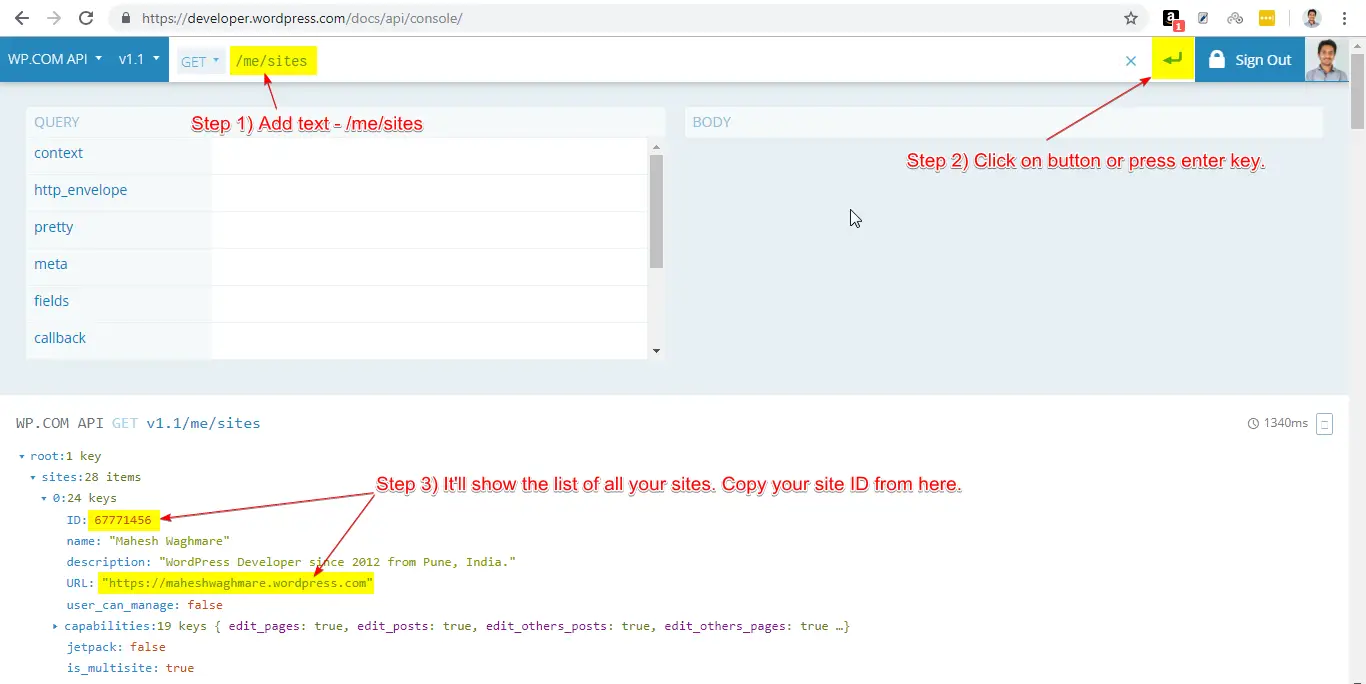
Step 7: Step 7:
Yup! Its not a step.
That’s it. Your first post successfully created!
Use below Complete Code snippet.Try this and let me know in comments.
The complete code snippet to create a post on wordpress.com is below:
Conclusion Conclusion
In above example you can create a post on wordpress.com with the help of get_access_key() and create_post() functions from above code snippet.
Note: use unique prefix for get_access_key and create_post() to avoid the conflict with existing function with same name.
Working with Rest API is pretty simple. Let’s try it and let me know in comments.