In this article we are going to see how to use WordPress Cron or WP Cron to register custom cron schedules and cron events.
You are going to learn:
See all WordPress Cron schedules? See all WordPress Cron schedules?
We can use the function wp_get_schedules()
which show all the exiting schedules something like below:
Add WordPress Cron Schedules Add WordPress Cron Schedules
In WordPress we have a filer cron_schedules
which allow us to add custom schedule.
We have see we can use the function wp_get_schedules() to see all the existing schedules.
In above example we have below schedules:
- hourly
- twicedaily
- daily
- weekly
But, We can use the cron_schedules to add our own custom schedules. Let’s add a every_ten_minutes schedule to perform some action.
Here, We have added a new schedule with 600 seconds interval means for every 10 minutes.
Add WordPress Cron Event Add WordPress Cron Event
We have register the custom schedule every_ten_minutes for every 10 minutes.
Now, Lets add the a custom schedule event or cron event.
We can register the schedule event with function wp_schedule_event().
See below code snippet:
Here, We have register the prefix_cron_hook schedule event. This scheduled event trigger for every 10 minutes.
But, We have to add a action which is same as our scheduled event.
We can use the same action prefix_cron_hook to perform some task. So, Lets add a sample task.
Add WordPress Cron Task Add WordPress Cron Task
We can use the function add_action() to perform our scheduled event with hook prefix_cron_hook.
See below code for reference:
Here, We have used function error_log() into our scheduled event task. So, Wherever our event prefix_cron_hook event trigger (every 10 minites) then our hook prefix_cron_hook called.
And our function prefix_cron_task() called which is hooked in action prefix_cron_hook.
In short; the string Called is added into the debug.log file (if WP_DEBUG & WP_DEBUG_LOG is true from wp-config.php file)
Test WordPress Cron Events? Test WordPress Cron Events?
We have an amazing WordPress plugin called WP Crontrol. The plugin WP Crontrol provide a user interface in which we can see the all registered scheduled and events.
Install and Activate the WP Crontrol plugin from WordPress back-end (wp-admin) Install and Activate the WP Crontrol plugin from WordPress back-end (wp-admin)
Follow below steps to install the WP Crontrol plugin.
- Go to Plugins > Add new
- Search for WP Crontrol
- Click on the Install now and then Activate button
See below screenshot for reference:

Install and Activate WP Crontrol with WP CLI Install and Activate WP Crontrol with WP CLI
Alternatively, If you have a WP CLI setup then you can install the WP Crontrol plugin with WP CLI command.
Use below command which install and activate the WP Crontrol plugin.
wp plugin install wp-crontrol --activate
If installed but not activate then use blow command to activate the WP Crontrol with command line
wp plugin activate wp-crontrol
See all WordPress Cron Schedules? See all WordPress Cron Schedules?
To see all the existing Cron schedules navigate to to Settings > Cron Schedules.
See below screenshot for reference:

Here, You can see our registered schedule every_ten_minutes which indicate the interval 600 seconds (Every 10 minutes).
See all WordPress Cron Events See all WordPress Cron Events
To see all cron event navigate to Tools > Cron Events.
See below screenshot for reference:

Here, You can see our scheduled event prefix_cron_hook which is recurrence (trigger for every) 10 minutes.
Manually Run the WordPress Cron Event Manually Run the WordPress Cron Event
Yes, The plugin WP Crontrol allow us to execute the Cron Event manually.
Just click on Run Now action link which appear blow the scheduled event on hover.
See below screenshot for reference:

Now, On click on Run now to and see the debug.log file which show you the string Called. You can see something below string:
[02-Jun-2020 13:57:08 UTC] Called
See below screenshot for reference:
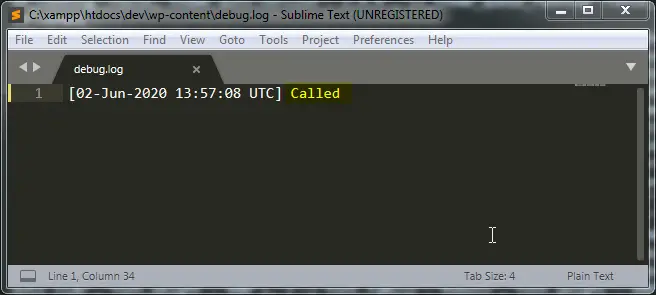
Here, For testing I have just used code error_log( ‘Called’ );. You can use your own task.
NOTE: In the debug.log file we cant see the logging data until we enable the debug log. We can use set constant DEBUG_LOG to true to enable the debug log.