We learn how to install the WP CLI. Now, Let’s create a simple WP CLI hello world program.
We are going to create a new plugin that contains the WP CLI hello world program.
Note: In this post, I’m focusing only on the main concepts. So, Not making the translation ready strings, not doing sanitization & escaping, also not adding class/function documentation, etc. Because I think beginners will not confuse the code.
E.g. For a beginner the code echo 'Hello';
is more understandable than esc_html_e( 'Hello', 'textdomain' );
.
Create Empty Plugin Create Empty Plugin
- Create a new folder
wordpress-examples
into plugins directory/wp-content/plugins/
- Create a file
wordpress-examples.php
and add the below code into it.
<?php /** Plugin Name: WordPress Examples */
- Now you can see our WordPress Examples plugin exists into the plugins list.

Now, Activate the plugin.

Register WP CLI Command Register WP CLI Command
Register PHP class WordPress_Examples_WP_CLI
e.g.
if ( ! class_exists( 'WordPress_Examples_WP_CLI' ) && class_exists( 'WP_CLI_Command' ) ) : class WordPress_Examples_WP_CLI extends WP_CLI_Command { } endif;
Here,
We have registered a new class WordPress_Examples_WP_CLI
and extend it with WP_CLI_Command
.
Add Example
Command Add Example
Command
Now let’s register the examples
. E.g.
WP_CLI::add_command( 'examples', 'WordPress_Examples_WP_CLI' );
Here,
We have used the function WP_CLI::add_command()
to register our examples
command.
The function WP_CLI::add_command()
accepts 2 parameters. First paramter is the command name. Which is examples
in our case.
And second is a callback class which is WordPress_Examples_WP_CLI
Add hello_world
sub command Add hello_world
sub command
public function hello_world( $args, $assoc_args ) { }
Here,
We have added a function hello_world()
with two parameters.
$args
contain the arguments.$assoc_args
contain the associate arguments.
We will learn more about these arguments in upcoming articles.
NOTE: that we have set the function scope as public. Any function within the WP CLI class considers as a subcommand.
In class WordPress_Examples_WP_CLI If we add another function with public scope then it is considered as a subcommand.
Echo/Print String Echo/Print String
WP_CLI::line('Hello World');
Here,
We have used the function WP_CLI::line()
to print the string Hello World
.
Testing Testing
Open command prompt/terminal. Go to your WordPress setup. I have set up WordPress in D:\xampp\htdocs\dev.test\
So, Execute the below commands:
cd D:\xampp\htdocs\dev.test
wp examples hello_world
You can see the output something like below:
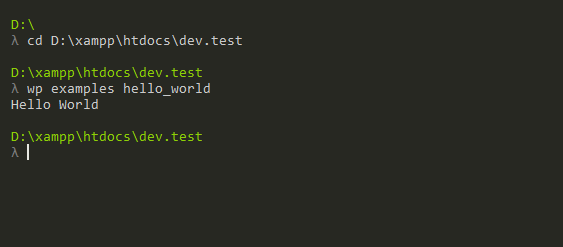
Here,
cd D:\xampp\htdocs\dev.test
– navigate to our WordPress setup.wp examples hello_world
– execute the command.
Complete Code Complete Code
if ( ! class_exists( 'WordPress_Examples_WP_CLI' ) && class_exists( 'WP_CLI_Command' ) ) : class WordPress_Examples_WP_CLI extends WP_CLI_Command { public function hello_world( $args, $assoc_args ) { WP_CLI::line('Hello World'); } } WP_CLI::add_command( 'examples', 'WordPress_Examples_WP_CLI' ); endif;
Understand Some Functions Understand Some Functions
WP_CLI::line()
– Print the message on the command prompt.WP_CLI::error()
– Print the message and terminate the execution of the CLI command.
Let’s see it with the below example:
public function hello_world( $args, $assoc_args ) { // Simple message. WP_CLI::line( 'Hello' ); // Error print here. WP_CLI::error( 'Testing the Error!' ); // This not print due to above error message. WP_CLI::line( 'World' ); }
Now, If we execute the command then the output is as below:
? wp examples hello_world Hello Error: Testing the Error!
Here, the string World
did not print because of the function WP_CLI::error()
print the message and terminate the window.
Github Repository Github Repository
You can also see the code with a well-written format on Github Repository WordPress Examples.
Create a first WP CLI Hello World Program in WordPress
Tweet