Minification is a process of reducing the size of JavaScript and CSS files by removing whitespace, comments, and other unnecessary characters.
While it can improve page load times, it can also make the code hard to read and debug.
In this article, we’ll explore how to avoid minification in Webpack and keep your code maintainable.
You are going to see:
What is Webpack? What is Webpack?
Webpack is a popular module bundler for JavaScript applications.
It allows you to organize your code into modules and dependencies, and then bundle them into a single file for deployment.
It also provides many optimization features, including minification.
Why Avoid Minification in Webpack? Why Avoid Minification in Webpack?
Minification can cause issues with debugging and development.
When the code is minified, variable and function names are changed, making it difficult to trace errors or understand what’s happening in the code.
Additionally, if you’re using a source map to debug your code, it may not match the minified code, making debugging even more challenging.
How to Avoid Minification in Webpack How to Avoid Minification in Webpack
Method #1 – mode Method #1 – mode
Use the mode
option The mode
option in Webpack allows you to set the mode of operation for the bundler.
When you set it to “development”, Webpack will not minify your code.
To set the mode, add the following line to your Webpack configuration file:
jsCopy codemodule.exports = {
mode: "development",
// rest of the configuration
}
Method #2 – devtool Method #2 – devtool
Use devtool
option The devtool
option in Webpack allows you to generate source maps for your code.
Source maps allow you to debug your original code instead of the minified code.
To generate source maps, add the following line to your Webpack configuration file:
jsCopy codemodule.exports = {
devtool: "source-map",
// rest of the configuration
}
Method #3 – uglifyjs-webpack-plugin Method #3 – uglifyjs-webpack-plugin
Use uglifyjs-webpack-plugin
with source-map
option If you still want to minify your code, you can use the uglifyjs-webpack-plugin
with the source-map
option.
This will generate a source map that matches the minified code, allowing you to debug it.
To use the plugin, install it with npm and add the following lines to your Webpack configuration file:
jsCopy codeconst UglifyJsPlugin = require('uglifyjs-webpack-plugin');
module.exports = {
// rest of the configuration
plugins: [
new UglifyJsPlugin({
sourceMap: true
})
]
}
Method #4 – optimization Method #4 – optimization
We can use the optimization
to avoid minification.
By default when executing the command:
webpack
Then, It builds the minified version of our source file into the destination file.
So, We can add the optimization
in the webpack.config.js file as below:
module.exports = function( env ) { ... return { ... optimization: { minimize: false }, } }
For example check the below screenshot in which, I have disabled the minification.
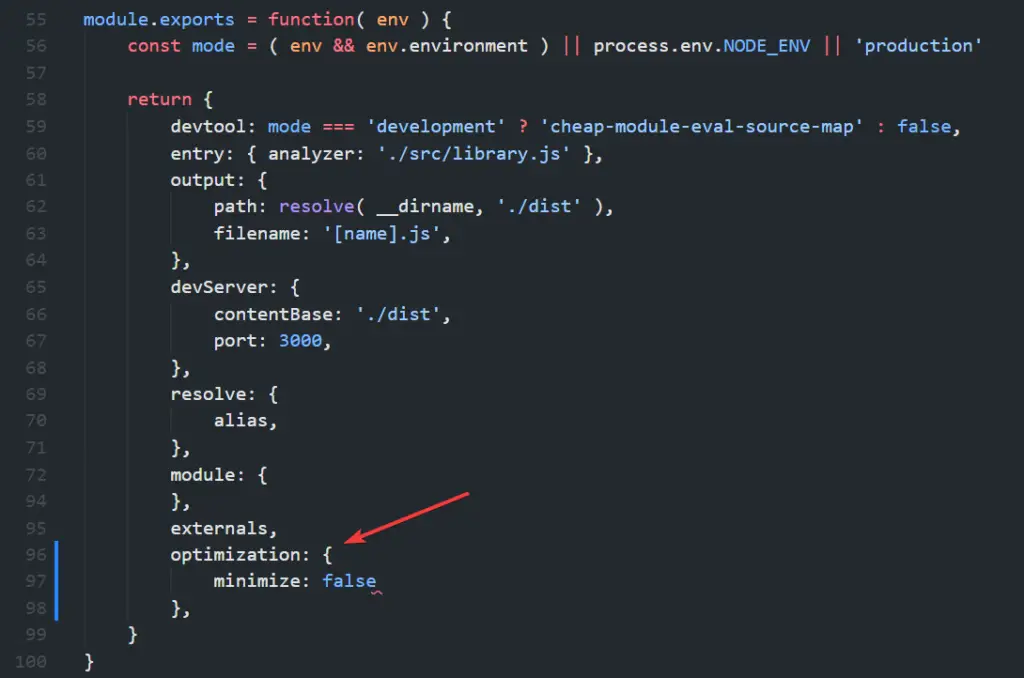
Conclusion Conclusion
Minification can be a helpful optimization technique for improving page load times, but it can also cause issues with debugging and development.
In this article, we’ve shown you how to avoid minification in Webpack and keep your code maintainable.
By using the mode
, devtool
, uglifyjs-webpack-plugin
, and optimization
options, you can easily control the minification process in your Webpack configuration.